How to Add a Switch Toggle Button in React Native
A toggle button is extremely useful when the user needs to give boolean input such as Yes or No. The switch component is used to create a switch/toggle button in react native. In this blog post, let’s check how to add a switch button to your react native project.
<Switch
trackColor={{ false: "#767577", true: "#81b0ff" }}
thumbColor="#f5dd4b"
ios_backgroundColor="#3e3e3e"
onValueChange={toggleSwitch}
value={isEnabled}
/>
The implementation of the toggle button is pretty straight as given above. The component works according to the value and onValueChange props. The trackColor, thumbColor and ios_backgroundColor props are used for styling the switch button.
Following is the complete example of react native switch button. Go through it for proper understanding.
import React, {useState} from 'react';
import {View, Switch, StyleSheet} from 'react-native';
const App = () => {
const [toggle, setToggle] = useState(false);
return (
<View style={styles.container}>
<Switch
trackColor={{false: 'gray', true: 'teal'}}
thumbColor="white"
ios_backgroundColor="gray"
onValueChange={value => setToggle(value)}
value={toggle}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#2c3e50',
},
});
export default App;
Have a look at the output of react native toggle button example.
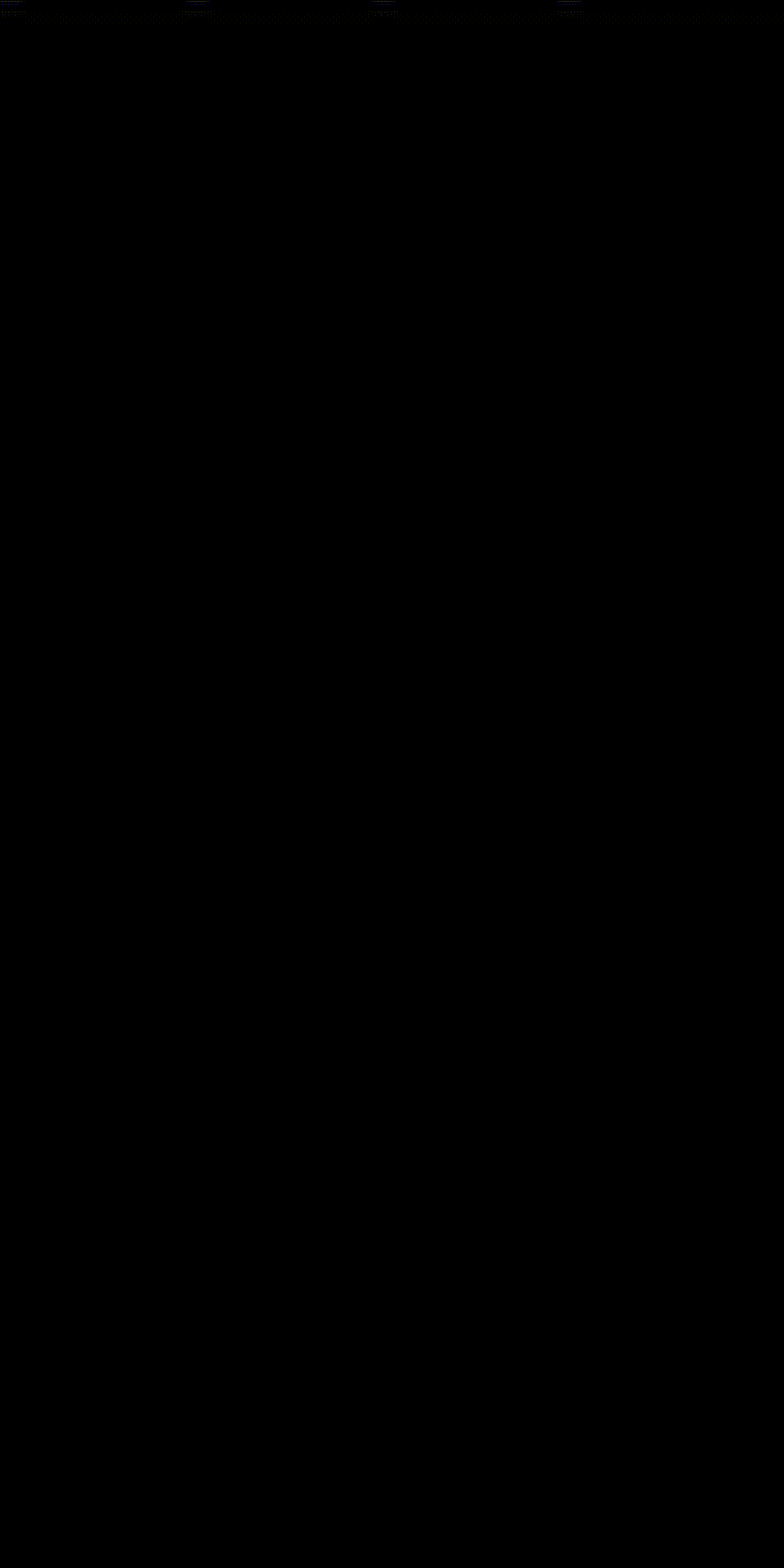
That’s how you add a switch button in react native. I hope this react native tutorial helped you!