How to Create Gradient Background in React Native
React Native doesn’t support gradient colors out of the box. Fortunately, there is a cool library named react native linear gradient which helps to create gradient patterns in react native apps. In this blog post, let’s see how to create a gradient background for any screen in react native
First of all, add the react native linear gradient library to your project using any of the following commands.
npm install react-native-linear-gradient --save
or
yarn add react-native-linear-gradient
For ios, run the following command from /ios folder to complete the installation.
pod install
That’s it for react native CLI users.
If you are using react native expo then you just need to execute the following command.
npx expo install expo-linear-gradient
In react native expo, you should import the library as given below.
import { LinearGradient } from 'expo-linear-gradient';
Coming to the execution part, you can use this library very easily. The basic usage of the library is as given below.
<LinearGradient colors={['#4c669f', '#3b5998', '#192f6a']}>
<View style={{width: 250, height: 250}} />
</LinearGradient>
Following is the code to create a full-screen gradient background in react native.
import React from 'react';
import {StyleSheet, Text} from 'react-native';
import LinearGradient from 'react-native-linear-gradient';
const App = () => {
return (
<LinearGradient
colors={['cyan', 'lightgreen']}
style={styles.container}
start={{x: 0, y: 0}}
end={{x: 1, y: 1}}>
<Text>Gradient Background</Text>
</LinearGradient>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
The output will be as given below:
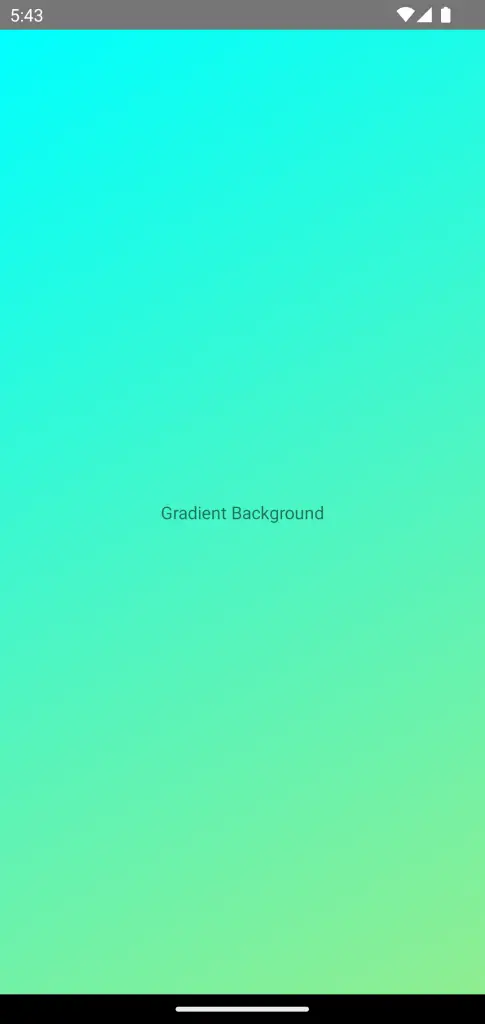
I hope this blog post will help you to create beautiful screens with gradient colors and patterns.