How to Detect React Native App is in Background
Sometimes, you may want to know whether your react native app is running background or running foreground to execute your tasks. You can use the AppState API from react native for this purpose.
If the AppState.currentState is active then it means your app is running in the foreground whereas if the AppState.currentState is background then it means your app is running in the background.
There’s another value inactive which is specifically for iOS. Inactive means your app is in a transition between foreground and background.
Following is the example code to detect whether the app is in the foreground or background in react native. This react native example is directly taken from react native official documentation.
import React, {useRef, useState, useEffect} from 'react';
import {AppState, StyleSheet, Text, View} from 'react-native';
const App = () => {
const appState = useRef(AppState.currentState);
const [appStateVisible, setAppStateVisible] = useState(appState.current);
useEffect(() => {
AppState.addEventListener('change', _handleAppStateChange);
return () => {
AppState.removeEventListener('change', _handleAppStateChange);
};
}, []);
const _handleAppStateChange = nextAppState => {
if (
appState.current.match(/inactive|background/) &&
nextAppState === 'active'
) {
console.log('App has come to the foreground!');
}
appState.current = nextAppState;
setAppStateVisible(appState.current);
console.log('AppState', appState.current);
};
return (
<View style={styles.container}>
<Text>Current state is: {appStateVisible}</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Following is the output.
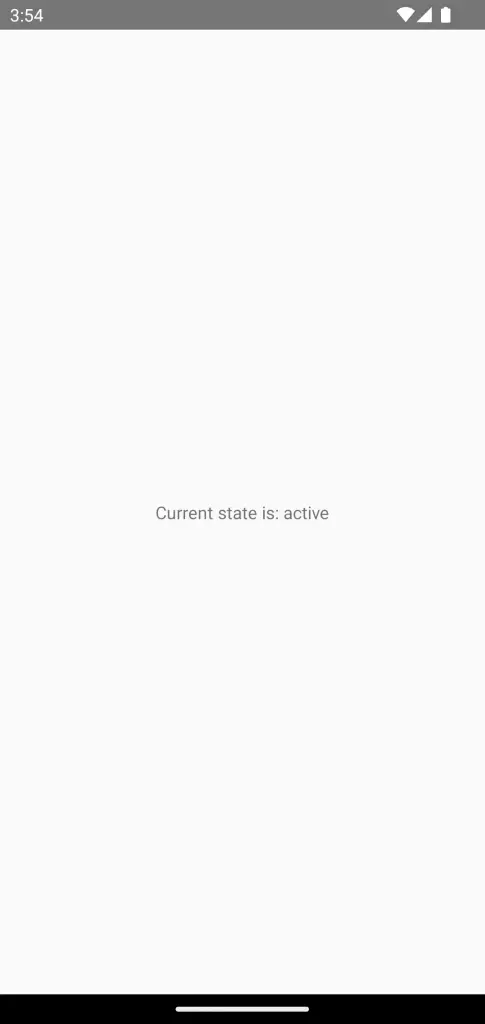
That’s how you detect whether your app is in the background or foreground in react native.
This is firing thrice when app is going to background instead of once
This is firing thrice when app is going to background instead of once